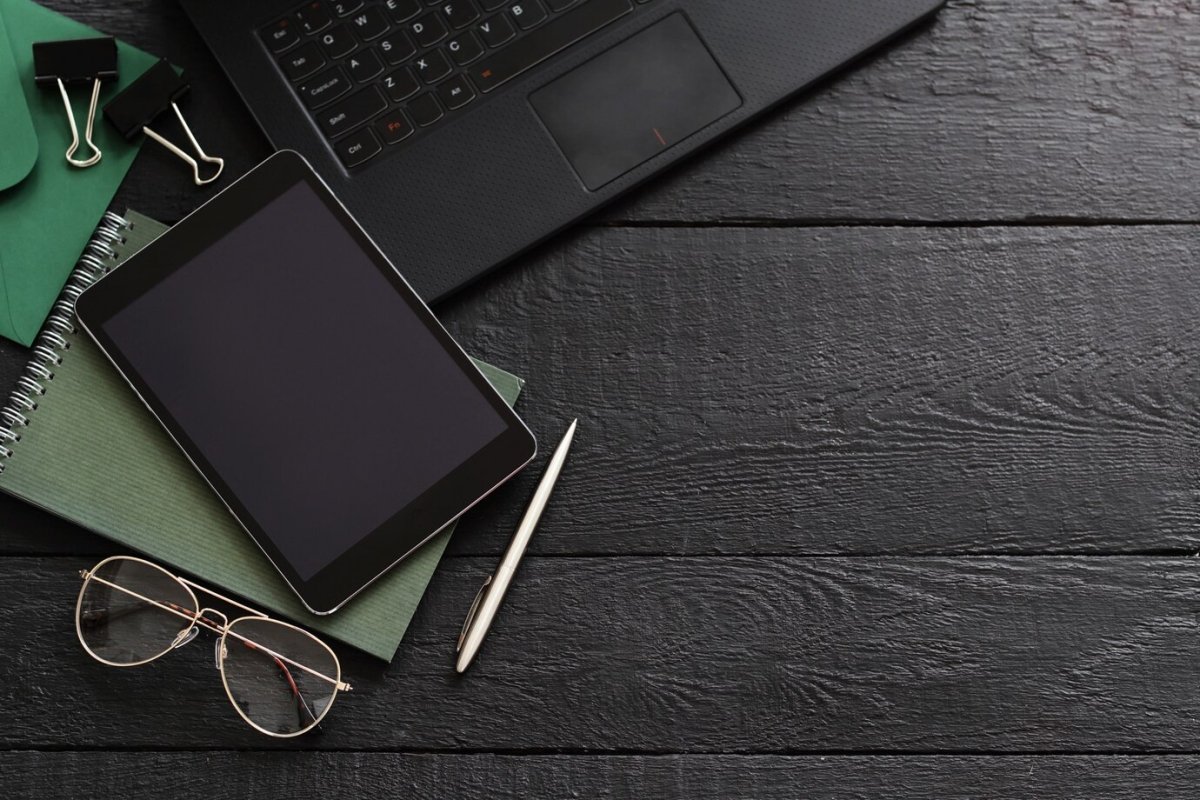
Développons votre site web !
Vous serez accompagné par un
expert en PHP, Symfony et Sylius
Plus de 10 ans d’expérience au service des e-commerçants. 🚀 (Et pas que !)
Développement
Créons ensemble des sites web performants et sur mesure, grâce à mon expertise en PHP, Symfony et Sylius.
Bonnes pratiques
Je conçois des sites web en respectant les meilleures pratiques et les standards Opquast ↗ pour une qualité optimale.
Boostons votre chiffre
J'optimise votre site pour convertir vos visiteurs en clients grâce à des solutions pensées pour l'acquisition et la performance.